Hello World
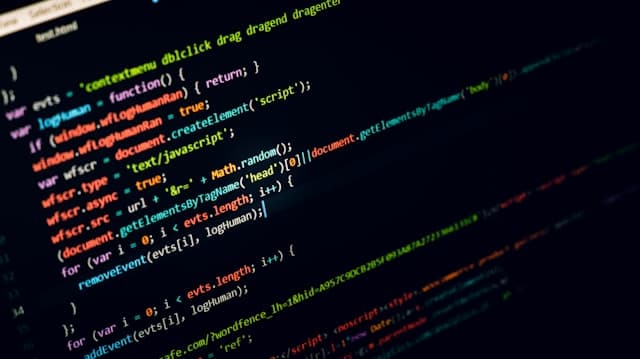
To start, you need to get GCC (GNUI Compiler Collection) running on your computer. I am not going to cover that here as I will only be able to speak for Mac users. If you don’t have C installed already, you can quickly search on google “How to install C on Windows” (Replace “Windows” with your operating system).
For the Text Editor/IDE, you can use VS code or Virtual Studio (if you are on window) or Vim. I will be using VS code myself. If you want to use VS code, you might need to install the C/C++ extension in the VS code marketplace.
Once everything has been set up, we can start writing our first helloword program.
Coding Starts Here
Start by creating a file called helloworld.c. In this file you can copy and paste the following code or type it if you prefer;
#include <stdio.h> int main() { printf("Hello World"); return 0; }
Code Breakdown
#include <stdio.h>
In this line of code, we are telling the preprocessor to include the content of <stdio.h> into our code. This enables us to be able to use the "printf" function and other useful functions we will look at in the future.
What is a "preprocessor"?
Here is how the life cycle of a C program looks like:
Basically, the first process in a C program's life cycle is the preprocessor. This takes our code and expands it by including the source code in our <stdio.h> file. To see this in action, let's create view how our preprocessed file looks like. To do this, open your terminal and change your directory to point to where the folder the C file is located and run the following command.
To change directory, the easiest way is to go to where the folder is located in your file manager and right click on the folder and click on copy(for macos) or copy as path(for windows). After that you can open your terminal and type "cd {paste the copied path here}". If you are not familiar with using the terminal, I will advice you to at least learn a couple of the common commands.
gcc -E helloworld.c -o helloworld.i
Don't worry too much about what this is doing for now. But basically, this is creating a file called helloworld.i in our directory and saving the output of the preprocessed file there. When you open this file, you will see lots of code in there, mostly what we didn't write. But when you scroll to the very end, you will see our code in there. The additional code is from our <stdio.h> file.
Hopefully, this gives you a basic idea of how the preprocessor works in our code.
You might also be wondering "what about the other steps in the life cycle?". So lets quickly go over those
Compiler
In this stage, our preprocessed source code from the helloworld.i file is converted to assembly. This is also where the error checking is done. Because C converts its source code to assembly and has high level abstraction/features, that is why most people consider C to be a "middle level" programming language. But on the other side, C is also called a "low level" programming language because due to its ability to convert to assembly, you can perform low level operations like writing directly to the computer's memory.
Assembler
The assembler then converts the assembly code to machine format (binary - which is 0's and 1')
Linker
The purpose of the linker is to produce a single executable file that can be run by the operating system. It makes sure that any reference to a function is resolved.
int main() {}
This is known as the main function. We will talk about functions later on. Just know that the main is known as the entry point to our program. This is the function that is called by the operating system.
printf("Hello World");
This is a function in C that basically allows us to print message in our console. We will see this working when we run the code.
return 0;
This tells the operating system if the program was run successfully. If you set it to 1, it mean it wasn't successful, and 0 means it was successful.
Now lets run our code
Once your terminal is opened and you have change directory to the folder containing the helloword.c file you can run the following command;
gcc helloworld.c -o helloworld
This should create an executable file called helloworld. We can then run this command to see our message
./helloword
[Ouput]: Hello World
Command breakdown
gcc
This is the compiler we are using to run our program. C has other compilers like CLang. We will be using gcc as that is widely used.
helloworld.c
This is our C file we want gcc to compile.
-o helloworld
This tells gcc to output our executable code into the helloworld file
This is an optional command. When we remove it, the full command becomes;
gcc helloworld.c
This works like before, just now the executable filename is going to be a.out.
./helloword
We use this command to open our executable file. If you are on windows, this will become;
.\helloword
Congratulation!!!, you've written your first C program.